React.js is one of the most popular and widely used JavaScript libraries. Among the many features React offers, two crucial hooks are often employed in the development process: Useref Vs Usestate.
These two hooks play distinct but equally vital roles in building dynamic, user-friendly web applications. In this article, we’ll delve into the meaning of both useRef and useState, explore their respective benefits, and guide you on when to useRef vs. useState.
Additionally, we’ll discuss how both of these hooks are incredibly beneficial for beginners in the world of React development.
Also Read: 27+ Best React Project Ideas For Beginners In 2023
What Is React useRef Vs useState
Before we delve into the comparison, let’s clarify the meaning and functionality of both useRef and useState.
React useRef Meaning
useRef is a hook in React that primarily deals with accessing and working with DOM elements directly. This hook allows developers to create a reference to a DOM element and persist it between renders. The primary use case useRef is accessing and modifying DOM properties or values, like focusing on input fields, triggering animations, or integrating third-party libraries that require direct access to DOM elements.
Meaning of React useState
On the other hand, useState is another essential hook in React, primarily used for managing and updating component states. The state is a fundamental concept in React, representing the dynamic data that can change over time, causing the UI to re-render when it does.
With useState, developers can manage state variables within functional components. It’s the go-to choice for managing application state, such as handling user input, toggling components, or updating content dynamically.
Major Benefits of Using useRef and useState
Good Benefits of useRef
- Direct DOM Manipulation: useRef empowers developers to manipulate DOM elements directly, making it invaluable for tasks that require precise control over the user interface.
- Optimizing Performance: By avoiding re-renders when working with DOM elements, useRef can help optimize the performance of your React application.
- Accessing Third-Party Libraries: Integration with third-party libraries, like charts or maps, often requires a direct reference to DOM elements, a task perfectly suited for useRef.
Benefits of useState
- Efficient State Management: useState simplifies the management of component state, ensuring smooth, dynamic updates without unnecessary re-rendering.
- User Input Handling: When handling user input, form data, or interactive UI components, useState is the go-to choice for tracking and managing changes.
- Enhanced Reusability: useState promotes the creation of reusable and encapsulated components, which is a cornerstone of maintainable and clean React code.
9+ Great Comparison Between React Useref Vs Usestate
Following are the major differences between useref vs usestate.
Aspect | useReffect | useState |
---|---|---|
Primary Purpose | Access and manipulate DOM elements directly | Manage and update component state |
Direct DOM Control | Yes | No |
Optimizing Performance | Yes, avoids unnecessary re-renders | May trigger re-renders |
User Input Handling | Not typically used for user input | Ideal for user input and form handling |
Integrating Third-Party Libraries | Helpful for direct DOM manipulation required by external libraries | Not the primary choice for this |
Suitable for Beginners | Yes, for understanding DOM manipulation | Yes, for learning state management and UI interaction |
Use Case Examples | Animations, focusing on input fields, third-party libraries | Toggling UI elements, handling form input, dynamic content updates |
Reusable Components | Typically not used for this purpose | Ideal for building reusable and encapsulated components |
Performance Of Useref Vs Usestate In 2023
In general, useRef is more performant than useState because it does not trigger re-renders when its value is updated. This is because refs are not part of the component state. On the other hand, updating the value of a state variable with useState triggers a re-render of the component.
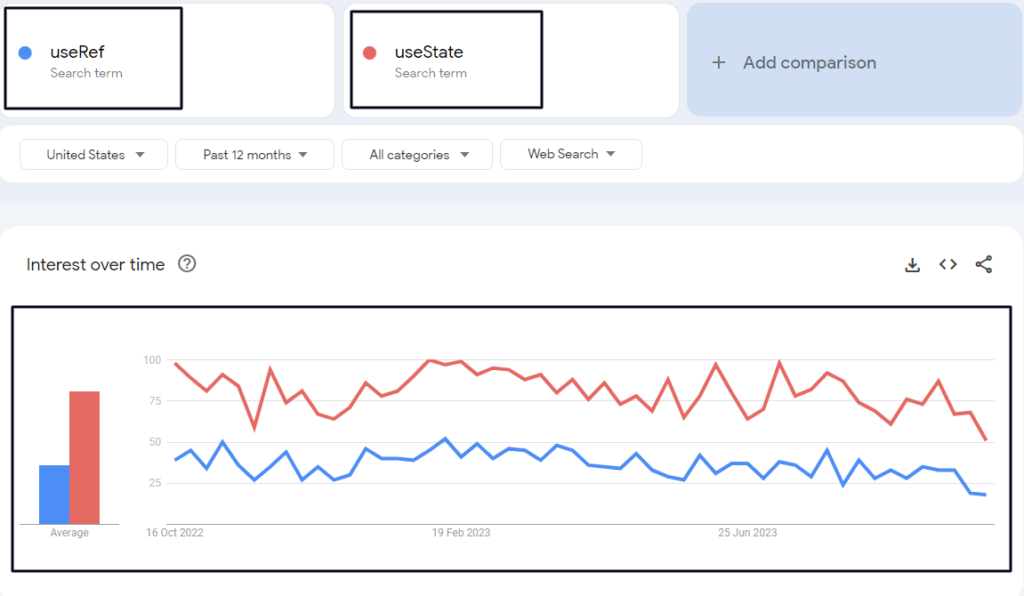
When to Use useRef vs useState
To choose between useRef vs useState, you need to consider the specific requirements of your project and how each hook aligns with those needs.
Let’s Know When to Use useRef
- Accessing DOM Elements: If your task involves direct manipulation of DOM elements, such as triggering animations, focusing on input fields, or working with third-party libraries, useRef is your ideal choice.
- Optimizing Performance: When you need to fine-tune your application’s performance by avoiding unnecessary re-renders, useRef can be a game-changer.
- Non-reliance on State Updates: Use useRef when your operation doesn’t depend on state updates. It’s perfect for actions that should remain unaffected by state changes.
Also, When to Use useState
- State Management: Whenever you need to manage component state, handle user input, or create dynamic, re-rendering UI components, useState should be your first pick.
- User-Interactive Elements: useState shines when your project involves user-driven changes, such as form handling, UI toggling, and data updates triggered by user actions.
- Reusable Components: For building reusable and encapsulated components that maintain their state, useState is the way to go.
Examples For Useref Vs Usestate
In this example, the inputRef ref is used to store a reference to the input element. The focus input function then uses the current property of the ref to focus the input element.
Here is an example of how to use useRef to access and manipulate the DOM:
import React, { useRef } from 'react';
const MyComponent = () => {
const inputRef = useRef(null);
const focusInput = () => {
inputRef.current.focus();
};
return (
<div>
<input type="text" ref={inputRef} />
<button onClick={focusInput}>Focus Input</button>
</div>
);
};
export default MyComponent;
Here is an example of how to use useState to manage component state
In this example, the useState hook is used to manage the count state variable. The incrementCount function then uses the setCount function to update the count state variable.
import React, { useState } from 'react';
const MyComponent = () => {
const [count, setCount] = useState(0);
const incrementCount = () => {
setCount(count + 1);
};
return (
<div>
<p>Count: {count}</p>
<button onClick={incrementCount}>Increment Count</button>
</div>
);
};
export default MyComponent;
Benefits for Beginners
Both useRef vs useState have their distinct benefits for beginners diving into React development.
Learning useRef
For beginners, grasping useRef opens the door to understanding the foundational concepts of direct DOM manipulation. This knowledge is crucial for building interactive web applications. It offers a hands-on approach to web development and provides insights into how web elements are controlled and animated.
Learning useState
useState is equally essential for beginners. It introduces the concept of state management, a fundamental skill in React. Learning useState helps beginners become proficient in managing and updating data dynamically, a skill transferable to a wide range of web development projects.
Conclusion
In the world of React development, understanding the differences between useRef vs useState is pivotal. These two hooks, while distinct in their purposes, are both essential for building robust and interactive web applications. To determine which one to use, consider the specific requirements of your project. useRef excels at direct DOM manipulation while useState is your go-to choice for efficient state management and user interactivity.
As a beginner, mastering both useRef vs useState will equip you with a versatile skill set that’s highly valuable in the world of web development. So, whether you’re manipulating DOM elements or managing component state, React has you covered with useReffect and useState.
FAQs
Why not to use useState in React?
More the number of usestate more bulkier the component will be and hence slow rendering time. Messy codebase and hence more chances of error and difficult to debug.
What is the problem with useState?
The problem with this is that the useState hook uses strict equality comparison to determine if it should trigger a re-render and doesn’t check if the properties of the object actually changed.
Should I always use useState?
If you have a single state either of a boolean, number, or string, use the useState hook.